일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | ||||||
2 | 3 | 4 | 5 | 6 | 7 | 8 |
9 | 10 | 11 | 12 | 13 | 14 | 15 |
16 | 17 | 18 | 19 | 20 | 21 | 22 |
23 | 24 | 25 | 26 | 27 | 28 |
- 반스 올드스쿨 VN000D3HY28
- 대림디움 대림바스플랜 PL-3070 탑카운터형 세면대/욕실/화장실/세면도기
- 리빙앤트리 펠리컨 박스 대
- 두칸 프릴 오버핏 후드티
- 미네 리빙다이닝 6인 원목 코너형 식탁세트(소파형)
- ipTIME 유무선공유기
- [제이앤제나] 허리에 무리없는 신생아부터 역류방지쿠션_앨리펀블루 역류방지쿠션
- 8052 블랙라인 정사각 세면기
- 오토비 AZ100TR 32G 전후방 FHD 2채널 트럭 화물차 전용 블랙박스 보증기간 2년 20m케이블 포함 후방 적외선 방수 AS 2년보장
- 러버메이드 벤티드 브루트(75L) 원형 컨테이너 리빙박스
- 네스파 다야몬드은박 후드티
- 내셔널지오그래픽 피너스 빅로고 라운드넥 긴팔 티셔츠 카본 블랙
- 23SS 스트라이프 오버핏 셔츠 723033 V2L30 9066
- 긍정
- 위드리빙 대용량 옷 수납 방수 바구니
- 22FW 로고 패치 포켓 체크 셔츠 2F000 03 M2359 F41
- ChatGPT
- 삼성 갤럭시워치5
- 굿아이템 양면오픈형 투명 접이식 리빙박스 폴딩박스 옷정리함 수납박스 펜트리수납함
- 샤오미 미밴드 7 스마트밴드
- 잡잡 NBR 요가매트 스탠다드 운동매트 10mm 퍼플
- 봄봄 골드라인 4023G 탑볼세면대 화이트골드 카운타 도기볼 카페세면대
- 코멧 접이식 투명 리빙박스 56L 대형 2개입
- 올리비아 스텐 세면대 세트
- 22FW 이바나 빈티지 체크 셔츠 더스트핑크 8054631
- 남쪽나무 세면대 세면대 세트 (스마트거울) 벽걸이세면대 이케아세면대 세면기
- 1개
- 톰브라운 명품톰브라운 23SS 더블페이스 니트 아우터형 포켓 셔츠 MJT291A 07545 415
- list #pop #remove #del #append #insert #list형 #파이썬 파이썬 기본 문법 #파이썬 list
- 더블탭스 22SS 정글 01 셔츠 WTAPS JUNGLE 01 GREIGE M
- Today
- Total
냥집사의 개발일지
C언어 - 구조체 (struct) (2) 본문
안녕하세요 저번 포스팅에 이어 구조체에 대해 더 정리해보겠습니다.
구조체의 활용 예제를 몇 가지 살펴볼 텐데요.
1. 배열 및 포인터를 구조체의 멤버 변수로 사용
2. 구조체를 구조체의 멤버 변수로 사용
1. 배열 및 포인터를 구조체의 멤버 변수로 사용
아래 예제는 이름을 배열로, 자기 소개말은 포인터로 구조체의 멤버 변수를 구성했습니다.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct profile{
char name[10];
int age;
char *introduction;
};
int main(){
struct profile me;
strcpy(me.name, "Jeff");
me.age = 20;
me.introduction = (char *)malloc(100 * sizeof(char));
printf("input the introduction : ");
gets(me.introduction);
printf("name : %s\n", me.name);
printf("age : %d\n", me.age);
printf("introduction : %s\n", me.introduction);
free(me.introduction);
return 0;
}
1. me라는 profile 구조체의 변수를 선언합니다.
2.strcpy란 문자열 복사 함수를 이용하여 name배열에 문자열을 복사합니다.
// strcpy(복사될 문자열, 복사할 문자열); (strcpy는 복사될 문자열의 포인터를 반환합니다.)
3. introduction 포인터에 100byte 동적 할당된 메모리를 가리키게 합니다.
4. gets 함수로 문자열을 입력받고 introduction 포인터에 할당합니다.
5. 아래 결과처럼 구조체 멤버 변수를 이용해 원하는 결과를 출력한 것을 확인 할 수 있습니다.
2. 구조체를 구조체의 멤버 변수로 사용
profile 구조체 안에 region 구조체가 멤버 변수로 있습니다.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct region{
char city [20];
char *district;
};
struct profile{
char name[10];
int age;
struct region re;
char *introduction;
};
int main(){
struct region re = {"Seoul", "Hongdae"};
struct profile me = {"Jeff", 20, re,"hello_world"};
struct profile you;
you = me;
printf("name : %s\n", you.name);
printf("age : %d\n", you.age);
printf("city : %s\n", you.re.city);
printf("district : %s\n", you.re.district);
printf("introduction : %s\n", you.introduction);
return 0;
}
1. 구조체는 구조체와 직접 대입이 가능합니다. (you = me)
2. 구조체 안에 구조체는 멤버 접근 연산자를 이용하여 접근할 수 있습니다. (you.re.city)
3. 아래 결과 처럼 출력하고자 하는 값이 출력되었음을 확인할 수 있습니다.
Tips 구조체는 구조체를 구조체에 복사할 수 있습니다.
다만, 주의해야 할 점은 구조체 멤버 변수 중 포인터 변수가 있다면
구조체 복사 시 얕은 복사가 되어 2개의 구조체 변수에서 같은 멤버 변수에 접근할 수 있는 문제가 발생합니다.
이와 같은 문제를 방지하기 위해 구조체를 복사한 뒤 포인터 멤버 변수만 따로 원본의 data를 복사하면 됩니다!
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct profile{
char name[10];
int age;
char *introduction;
};
int main(){
struct profile me = {"Jeff", 20, NULL};
me.introduction = (char *)malloc(100 * sizeof(char));
struct profile you;
strcpy(me.introduction, "hello_Jeff");
you = me;
strcpy(me.introduction, "hello_Alana");
printf("name : %s\n", you.name);
printf("age : %d\n", you.age);
printf("introduction : %s\n", you.introduction);
free(me.introduction);
free(you.introduction);
return 0;
}
you에 me를 대입한 뒤 me의 introduction에 "hello_Alana" 문자열을 대입했는데
아래 결과를 보니 you의 introduction이 수정된 것을 확인할 수 있습니다.
이는 me&you의 introduction 포인터가 같은 주소를 가리키고 있기 때문입니다. (얕은 복사)
또한 같은 주소가 이중으로 free 되는 문제가 발생합니다.
free가 이중으로 될 시 process는 종료됩니다!!
아래 예제에서는 you의 introduction을 동적 할당해주고 me의 introduction의 data를 strcpy로 복사하였습니다. (깊은 복사)
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct profile{
char name[10];
int age;
char *introduction;
};
int main(){
struct profile me = {"Jeff", 20, NULL};
me.introduction = (char *)malloc(100 * sizeof(char));
struct profile you;
strcpy(me.introduction, "hello_Jeff");
you = me;
you.introduction = (char *)malloc(strlen(me.introduction) + 1);
strcpy(you.introduction, me.introduction);
strcpy(me.introduction, "hello_Alana");
printf("name : %s\n", you.name);
printf("age : %d\n", you.age);
printf("introduction : %s\n", you.introduction);
free(me.introduction);
free(you.introduction);
return 0;
}
아래 결과처럼 me의 introduction이 you의 introduction에 영향을 주지 않고 독립적이라는 것을 확인할 수 있습니다.
저번 포스팅에 이어 구조체에 대해 정리해보았습니다.
구조체 멤버 변수로 배열, 포인터, 구조체를 선언하는 방법, 구조체에 구조체를 복사할 때 주의점 등
을 알아보았는데요. 이번 포스팅이 구조체를 이해하는데 많은 도움이 되었으면 좋겠습니다~
다음 포스팅에서 만나요~
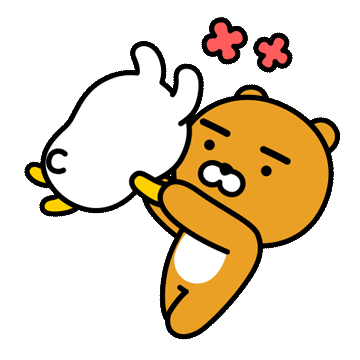
'C언어' 카테고리의 다른 글
C언어 - 공용체,열거형,typedef (union,enum,typedef) (0) | 2022.09.26 |
---|---|
C언어 - 구조체 포인터 & 구조체 배열 (struct pointer & struct array) (0) | 2022.09.25 |
C언어 - 구조체 (struct) (1) | 2022.09.23 |
C언어 - 메모리 동적 할당 (문자열) (0) | 2022.09.22 |
C언어 - 메모리 동적 할당 (calloc, realloc) (0) | 2022.09.21 |