일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | |||
5 | 6 | 7 | 8 | 9 | 10 | 11 |
12 | 13 | 14 | 15 | 16 | 17 | 18 |
19 | 20 | 21 | 22 | 23 | 24 | 25 |
26 | 27 | 28 | 29 | 30 | 31 |
- 러버메이드 벤티드 브루트(75L) 원형 컨테이너 리빙박스
- 굿아이템 양면오픈형 투명 접이식 리빙박스 폴딩박스 옷정리함 수납박스 펜트리수납함
- 내셔널지오그래픽 피너스 빅로고 라운드넥 긴팔 티셔츠 카본 블랙
- 위드리빙 대용량 옷 수납 방수 바구니
- 대림디움 대림바스플랜 PL-3070 탑카운터형 세면대/욕실/화장실/세면도기
- 리빙앤트리 펠리컨 박스 대
- 22FW 로고 패치 포켓 체크 셔츠 2F000 03 M2359 F41
- 반스 올드스쿨 VN000D3HY28
- 더블탭스 22SS 정글 01 셔츠 WTAPS JUNGLE 01 GREIGE M
- 22FW 이바나 빈티지 체크 셔츠 더스트핑크 8054631
- 오토비 AZ100TR 32G 전후방 FHD 2채널 트럭 화물차 전용 블랙박스 보증기간 2년 20m케이블 포함 후방 적외선 방수 AS 2년보장
- 8052 블랙라인 정사각 세면기
- 네스파 다야몬드은박 후드티
- list #pop #remove #del #append #insert #list형 #파이썬 파이썬 기본 문법 #파이썬 list
- 올리비아 스텐 세면대 세트
- 코멧 접이식 투명 리빙박스 56L 대형 2개입
- 두칸 프릴 오버핏 후드티
- 샤오미 미밴드 7 스마트밴드
- 23SS 스트라이프 오버핏 셔츠 723033 V2L30 9066
- ipTIME 유무선공유기
- 남쪽나무 세면대 세면대 세트 (스마트거울) 벽걸이세면대 이케아세면대 세면기
- [제이앤제나] 허리에 무리없는 신생아부터 역류방지쿠션_앨리펀블루 역류방지쿠션
- 긍정
- ChatGPT
- 봄봄 골드라인 4023G 탑볼세면대 화이트골드 카운타 도기볼 카페세면대
- 톰브라운 명품톰브라운 23SS 더블페이스 니트 아우터형 포켓 셔츠 MJT291A 07545 415
- 잡잡 NBR 요가매트 스탠다드 운동매트 10mm 퍼플
- 삼성 갤럭시워치5
- 1개
- 미네 리빙다이닝 6인 원목 코너형 식탁세트(소파형)
- Today
- Total
냥집사의 개발일지
C언어 - 구조체 포인터 & 구조체 배열 (struct pointer & struct array) 본문
안녕하세요 오늘은 구조체 포인터와 구조체 배열에 대해 정리해보겠습니다.
1. 구조체 포인터 와 '->'연산자
다들 아시다 시피 당연히 포인터 변수가 구조체를 가리킬 수 있겠죠.
이때 구조체 포인터 변수는 '->' 연산자를 통해 구조체 멤버 변수에 접근할 수 있습니다.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct profile{
char name[10];
int age;
char *introduction;
};
int main(){
struct profile me = {"Jeff", 20, NULL};
me.introduction = (char *)malloc(100 * sizeof(char));
strcpy(me.introduction, "hello_Jeff");
struct profile *me_pointer = &me;
printf("name : %s\n", (*me_pointer).name);
printf("age : %d\n", me_pointer->age);
printf("introduction : %s\n", me_pointer->introduction);
free(me.introduction);
return 0;
}
1. profile 구조체 'me'를 가리키는 me_pointer 포인터를 선언합니다.
2. 위의 코드에서 알 수 있듯이 구조체가 멤버 변수에 접근할 때는 '.' 멤버 접근 연산자를 사용하고
구조체 포인터가 멤버 변수에 접근할 때는 '->' 연산자를 사용합니다.
3. 아래 결과 처럼 의도한 데이터가 출력되었음을 확인할 수 있습니다.
2. 구조체 배열
아래 코드는 구조체 배열을 초기화하고 함수의 매개변수로 받아 출력해보는 예제입니다.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct profile{
char name[10];
int age;
char *introduction;
};
void print_struct_arr(struct profile *friends, int size){
for(int i=0; i<size; i++){
printf("name : %s\n", (friends+i)->name);
printf("age : %d\n", (friends+i)->age);
printf("introduction : %s\n", (friends+i)->introduction);
printf("\n");
}
}
int main(){
struct profile friends[5] = {
{"Jeff", 20, "hello_Jeff"},
{"Electra", 19, "hello_Electra"},
{"Alana", 21, "hello_Alana"},
{"John", 19, "hello_John"},
{"Steven", 18, "hello_Steven"}
};
print_struct_arr(friends,sizeof(friends)/sizeof(struct profile));
return 0;
}
1. friends라는 profile 구조체 5개 요소를 가진 구조체 배열을 선언하고 초기화합니다.
2. print_struct_arr 함수에 매개변수로 구조체 배열을 받고 '->' 연산자를 이용해 멤버 변수들을 출력합니다.
3. 아래 결과 처럼 의도한 data들이 정상적으로 출력됐음을 확인할 수 있습니다.
3. 자기 참조 구조체
자료구조를 공부하면 가장 먼저 배우는 연결 리스트가 바로 자기 참조 구조체의 대표적인 예시인데요.
아래 코드를 같이 살펴보시죠~
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct list{
int num;
struct list *next;
};
void print_list(struct list *current){ // current : current point of list
while(current != NULL){
printf("%d ",current->num);
current = current->next;
}
}
int main(){
struct list a = {1,0}, b = {2,0}, c = {3,0};
struct list *head; //head : intital point of list
head = &a;
a.next = &b;
b.next = &c;
print_list(head);
return 0;
}
1. 구조체 list의 변수 3개 a, b, c를 선언하고 구조체 list의 포인터 변수 head를 선언합니다.
2. head는 a를, a는 b를, b는 c를 가리킵니다.
3. print_list 함수로 list의 멤버 변수 num를 순회하며 출력합니다. (아래 그림 참조)
head가 current에 할당되며 current도 a를 가리킵니다.
current의 current의 next 즉, a의 next가 할당되며 current는 b를 가리키게 됩니다.
current의 current의 next 즉, b의 next가 할당되며 current는 c를 가리키게 됩니다.
이때 c의 next는 NULL이므로 루프 문을 빠져나오게 됩니다.
최종적으로 current 포인터가 list 변수들을 순회하며 num 멤버 변수를 정상적으로 출력한 것을 확인할 수 있었습니다.
오늘은 구조체 포인터와 구조체 배열에 대해 정리해보았습니다!!
구조체에 대해 더 궁금하신 분들은 저번 포스팅도 참고해주세요~
2022.09.23 - [C언어] - C언어 - 구조체 (struct)
C언어 - 구조체 (struct)
안녕하세요 오늘은 구조체에 대해 알아보겠습니다~ 지금까지는 기본 자료형(int, double, char etc.)등으로만 코드를 구성했다면 오늘은 사용자 정의 자료형 즉, user의 필요에 따른 자료형을 struct를
leggo-fire.tistory.com
2022.09.24 - [C언어] - C언어 - 구조체 (struct) (2)
C언어 - 구조체 (struct) (2)
안녕하세요 저번 포스팅에 이어 구조체에 대해 더 정리해보겠습니다. 구조체의 활용 예제를 몇 가지 살펴볼 텐데요. 1. 배열 및 포인터를 구조체의 멤버 변수로 사용 2. 구조체를 구조체의 멤버
leggo-fire.tistory.com
그럼 다음에 만나요~
감사합니다. 좋은 하루 보내세요!!
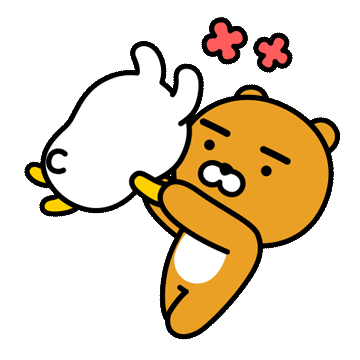
'C언어' 카테고리의 다른 글
C언어 - 공용체,열거형,typedef (union,enum,typedef) (0) | 2022.09.26 |
---|---|
C언어 - 구조체 (struct) (2) (0) | 2022.09.24 |
C언어 - 구조체 (struct) (1) | 2022.09.23 |
C언어 - 메모리 동적 할당 (문자열) (0) | 2022.09.22 |
C언어 - 메모리 동적 할당 (calloc, realloc) (0) | 2022.09.21 |